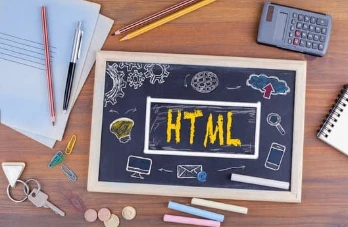
How to prepare for an HTML
and CSS interview?
HTML and CSS are two core technologies in web development. HTML is used to create the structure of a webpage, while CSS is responsible for its design and layout. Together, they form the foundation of any website.
In interviews, these technologies are tested because they are essential skills for web developers. Companies want to know if candidates can build and style web pages properly. Understanding HTML and CSS shows that you have a good grasp of how the front-end of a website works, making these questions common in technical interviews.
Basic HTML Interview Questions
-
What is HTML and why is it used?
What are the key differences between HTML4 and HTML5?
- HTML5 supports audio and video without requiring plugins.
- New semantic elements like <article>, <section>, and <nav> improve the structure of webpages.
- HTML5 allows for local storage using web storage (localStorage and sessionStorage).
How do you create a hyperlink in HTML?
How do you insert an image in an HTML page?
HTML (HyperText Markup Language) is the standard language used to create and structure content on the web. It organizes text, images, and links into meaningful sections so that browsers can display them properly. HTML forms the backbone of any webpage.
HTML5 is the latest version of HTML, introducing new elements and features. Some key differences include:
To create a hyperlink in HTML, you use the <a> tag. For example:
html
<a href="https://www.example.com">Visit Example>
The href attribute contains the link's destination, and the text between the tags is clickable.
You can insert an image in HTML using the <img> tag. Example:
html
<img src="image.jpg" alt="Description of Image">
The src attribute specifies the image file, and the alt attribute provides alternative text in case the image doesn't load.
Basic CSS Interview Questions
What is CSS and why is it important in web design?
What are the different types of CSS (inline, internal, external)?
-
How do you use CSS to center content on a webpage?
What is the box model in CSS?
CSS (Cascading Style Sheets) is used to control the appearance and layout of a webpage. It helps separate content from presentation, allowing developers to apply styles like colors, fonts, and spacing across multiple pages. CSS is essential in making a website visually appealing and easy to navigate.
Inline CSS: Applied directly to an HTML element using the style attribute. Example:
html
<p> style="color: blue;">This is blue text.<p>
Internal CSS: Defined within the <div> tag in the head section of an HTML file.Example
html
<style>
p { color: green; }
<style>
External CSS: Stored in a separate .css file and linked to the HTML file. Example:
html
<link rel="stylesheet" href="styles.css">
There are several ways to center content in CSS:
For horizontal centering of block elements, you can use margin: 0 auto;. Example:
css
div {
width: 50%;
margin: 0 auto;
}
For vertically and horizontally centering an element inside a parent, you can use Flexbox. Example:
css
.parent {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
The CSS box model defines the space an element takes up on a webpage. It consists of:
- Content: The actual content inside the box (text, images, etc.).
- Padding: The space between the content and the border.
- Border: A line surrounding the padding.
- Margin: The space outside the border that separates the element from others.
Understanding the box model is key to managing element spacing and layout in CSS.
Advanced HTML Interview Questions
What is semantic HTML, and why is it important?
What is the difference between section, div, and article elements?
- <section>: Used to define sections of content that are related or share a common theme. It's often used to group content logically, such as different parts of an article or page.
- <div>: A generic container with no semantic meaning. It's typically used for grouping elements together for styling purposes with CSS or JavaScript, but it doesn't tell the browser anything about the content.
- <article>: Represents self-contained, independent content. It can be used for blog posts, news articles, or any other section of content that makes sense on its own, even outside the context of the page.
How do you handle forms in HTML?
Semantic HTML refers to using HTML elements that have clear meaning and purpose. For example, using <header>, <footer>, <nav>, and article instead of generic elements like div. This makes the structure of the page more understandable for both developers and browsers. It also improves accessibility and search engine optimization (SEO) as search engines can better understand the content of a page.
Forms are handled in HTML using the