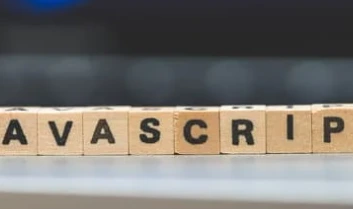
Lexical Environment in JavaScript
In JavaScript a lexical environment is the space where variables and functions are stored and can be accessed when the code runs. It serves as a container for all the identifiers (like variables and function declarations) within a specific scope.
Every time JavaScript code is executed whether globally or within a function a new lexical environment is created. This environment tracks the identifiers defined in that block of code. Each environment also maintains a connection to its parent environment allowing it to look up variables that are not found locally ensuring smooth variable management. This is crucial in JavaScript's scope system where variables are resolved based on their location within the code.
Understanding Lexical Environment
A lexical environment in JavaScript is the structure that holds variables functions and references used during code execution. It consists of two main parts:
- Environment Record: This part stores variables function declarations and bindings within a particular scope. It's like a storage unit for all the data that is accessible in that scope. For example variables defined within a function or block are kept in the environment record of that lexical environment.
(DEV Community )( In Plain English ). - Outer Environment Reference: This is a link to the parent environment which allows JavaScript to resolve variables that are not found in the current environment by looking up the scope chain. This connection ensures that if a variable is not available locally it can still be accessed from the outer scopes(
DEV Community )( DEV Community ).
Components of a Lexical Environment
- Environment Record: It stores all the variables and functions defined in the current scope. Every new scope creates a new environment record to keep track of all identifiers.
- Outer Environment Reference: This links to the parent lexical environment which allows the program to search for variables in broader scopes when they are not found in the local one.(
DEV Community ).
Types of Lexical Environments
- Global Lexical Environment: The global lexical environment is created as soon as the JavaScript code starts running. It holds all globally defined variables and functions. These are available throughout the entire code.(
DEV Community ). - Local Lexical Environment: A local lexical environment is created every time a function is called or a block of code is executed (like inside an if statement). Variables declared inside the function or block are stored in this local environment and are not accessible outside it.(
MDN Web Docs )( In Plain English ).
Example: Global and Local Environments in Action
let globalVar = 'I am global';
function exampleFunction() {
let localVar = 'I am local';
console.log(globalVar); // Accesses global variable
}
exampleFunction();
In this example the globalVar
is stored in the global lexical environment accessible anywhere in the code. The localVar
is stored in the local lexical environment of exampleFunction
and is only accessible within that function. The function can still access globalVar
because of the outer environment reference to the global environment​(
MDN Web Docs
)(
DEV Community
).
How Lexical Scoping Works
Lexical scoping in JavaScript refers to how variable names are resolved in nested functions based on where they are physically written in the code. JavaScript uses this "lexical" structure to determine which variables are accessible at any given point in the code. This means that the availability of variables is determined by their location within the source code not where or when functions are executed (
MDN Web Docs
)(
DEV Community
).
MDN Web Docs )( In Plain English ).
Example with Nested Functions
function outerFunction() {
let outerVar = 'I am outside!';
function innerFunction() {
let innerVar = 'I am inside!';
console.log(outerVar); // Can access outerVar
}
innerFunction();
console.log(innerVar); // Error: innerVar is not defined
}
outerFunction();
In this example
The outerFunction
has a variable outerVar
in its lexical environment.
The innerFunction
has its own lexical environment but it can still access outerVar
because of lexical scoping. However, innerVar
is not accessible outside innerFunction
because it exists only in that specific environment(
DEV Community
).
This behavior shows how variables are resolved based on where they are declared, demonstrating the core concept of lexical scoping.
Scope Chain and Lexical Environment
The scope chain in JavaScript is the mechanism that JavaScript uses to look up variables when executing a function. It determines the hierarchy of lexical environments that JavaScript must go through to resolve variables. Every time a function is called a new lexical environment is created for that function and the scope chain allows JavaScript to search through all the outer lexical environments to find variables that aren't available in the current scope(
In Plain English
)(
DEV Community
).
How the Scope Chain Works
When JavaScript needs to access a variable:
- It first looks in the local lexical environment where the variable is being referenced (e.g. inside a function or block).
- If the variable is not found it goes up one level to the outer lexical environment following the outer environment reference.
- This process continues up the chain until it reaches the global environment which is the highest level of scope in a JavaScript program​(
In Plain English ).
Multiple Lexical Environments in the Scope Chain
In JavaScript, multiple lexical environments can coexist due to the nesting of functions or blocks. These environments are connected through the scope chain, allowing inner functions to access variables from their outer lexical environments.
Example:
javascript
Copy code
let globalVar = 'I am global';
function outerFunction() {
let outerVar = 'I am in the outer function';
function innerFunction() {
let innerVar = 'I am in the inner function';
console.log(globalVar); // Accesses variable from global scope
console.log(outerVar); // Accesses variable from outer function
}
innerFunction();
}
outerFunction();
In this example:
- The global lexical environment contains
globalVar
. - The outerFunction creates its own lexical environment containing
outerVar
. - Inside
innerFunction
, the JavaScript engine first looks forouterVar
within the inner lexical environment. Since it doesn't find it there, it follows the scope chain to the outer lexical environment ofouterFunction
. It also accessesglobalVar
by following the chain all the way up to the code global lexical environment(
DEV Community )(
In Plain English )( DEV Community ).
This interaction between multiple lexical environments through the scope chain is a key feature of JavaScript's scoping system, enabling nested functions to access variables from their parent environments.
Lexical Environment and Closures
A closure in JavaScript is a function that has access to its own scope the scope of its parent function and the global scope. Closures are created whenever a function is created allowing that function to retain access to the variables from its lexical environment even after the outer function has finished executing(
DEV Community
)(
MDN Web Docs
).
Relationship Between Closures and Lexical Environment
Closures maintain references to the lexical environment in which they were created. This means that when an inner function (closure) is returned from an outer function, it "remembers" the variables in the outer function's scope, even after the outer function has completed. This is possible because the lexical environment of the outer function persists in memory, thanks to the closure(
DEV Community
)(
MDN Web Docs
).
Code Example:Javascript
function outerFunction() {
let outerVar = 'Outer';
return function innerFunction() {
console.log(outerVar); // Accesses outerVar from the outer lexical environment
};
}
const closure = outerFunction();
closure(); // Output: Outer
In this example
- The
outerFunction
creates a variableouterVar
and returns theinnerFunction
. - Even after
outerFunction
has finished execution, the returnednnerFunction
i (closure) retains access toouterVar
because of the closure, which keeps a reference to its outer lexical environment(
DEV Community )( DEV Community ).
This is the essence of closures in JavaScript: functions that "carry" their lexical environment along with them, allowing access to variables that otherwise would not be available after the outer function completes.
Common Mistakes with Lexical Environments
Lexical environments are central to how JavaScript handles variable scoping, but they can lead to reference errors and unexpected variable behavior when misunderstood. These issues often arise due to confusion about how JavaScript resolves variables or due to scoping quirks like the use of var
, let
, and const
.
- Reference Errors:
- Unexpected Variable Access with
var
- Scoping Issues in Loops
- Closures and Memory Leaks
OOne common mistake with lexical environments is attempting to access a variable before it is declared, resulting in a reference error. This often happens in cases where variables are declared with let or const, as they are block-scoped and are not accessible before their declaration due to the temporal dead zone.
Example:
javascript
Copy code
console.log(myVar); // ReferenceError: myVar is not defined
let myVar = 10;
In this case, JavaScript throws a reference error because the variable myVar is accessed before it's declared. Even though myVar exists in the lexical environment, it's not available for use until the line where it's declared due to the temporal dead zone(
DEV Community
)(
MDN Web Docs
).
Example:
javascript
Copy code
console.log(myVar); // Output: undefined
var myVar = 10;
In this example, myVar is hoisted, so the console.log does not throw an error, but it outputs undefined instead of the expected value. This can lead to confusing bugs when developers expect myVar to be fully initialized(
DEV Community
)(
In Plain English
).
A frequent bug in JavaScript comes from misunderstanding how variables declared with var behave in loops. Since var is function-scoped, it does not create a new lexical environment for each loop iteration, leading to unexpected results.
Example:
javascript
Copy code
for (var i = 0; i < 3; i++) {
setTimeout(function() {
console.log(i); // Output: 3, 3, 3
}, 100);
}
Here, developers might expect the values 0, 1, and 2, but var causes the value of i to be shared across all iterations. When the setTimeout function runs, the value of i is 3 because the loop has finished. Using let instead would fix this issue, as let creates a new lexical environment for each iteration(
DEV Community
)(
DEV Community
).
Closures are powerful, but they can lead to memory leaks if not handled properly. When a closure maintains references to variables in its outer lexical environment, it can prevent those variables from being garbage-collected, leading to inefficient memory use.
Example:
javascript
Copy code
function createClosure() {
let bigData = new Array(10000).fill('data');
return function() {
console.log(bigData[0]); // Closure retains reference to bigData
};
}
let closure = createClosure();
closure();
In this case, the closure retains access to the large bigData array even after createClosure has completed, leading to a potential memory leak if bigData is no longer needed(
DEV Community
)(
DEV Community
).
By understanding these common pitfalls, developers can avoid frustrating bugs and write more predictable, efficient JavaScript code.
Best Practices for Managing Lexical Environments
Managing lexical environments effectively in JavaScript ensures better readability, maintainability, and fewer scoping issues in your code. Here are some best practices to keep in mind:
- Use
let
andconst
for Block-Scoped Variables. - This creates a new lexical environment for each loop iteration, avoiding the common pitfalls of
var
(
DEV Community ) - Limit the Use of Global Variables.
- This avoids unnecessarily exposing variables to the global environment( DEV Community )
- losures for Controlled Variable Access C
- This pattern allows controlled access to count without exposing it directly(
DEV Community )(
DEV Community ). - Modularize Code to Improve Scope Management
- This keeps each function's lexical environment self-contained, making it easier to debug and maintain(
DEV Community ). - Avoid Hoisting Pitfalls
- This helps avoid the confusion caused by hoisting(
MDN Web Docs ). - Use Strict Mode .
- This helps avoid potential bugs and makes your code more robust(
DEV Community ). - Clear Unused Variables .
Avoid using var: Since var is function-scoped, it can lead to unexpected behavior, especially in loops and blocks. Using let and const ensures that variables are scoped properly within the block and reduces the chances of accidental overwrites or leaks.
javascript
Copy code
for (let i = 0; i < 3; i++) {
console.log(i); // Outputs 0, 1, 2 as expected
}
Minimize global scope pollution: Global variables are accessible everywhere, which can lead to accidental changes and harder-to-debug issues. Encapsulate variables within functions or blocks to limit their scope and improve maintainability.
javascript
Copy code
Copy code
function calculate() {
const value = 10;
// Use the variable locally
}
Leverage closures when necessary: Closures are useful for encapsulating functionality and data, but should be used judiciously to avoid memory leaks. When using closures, be mindful of which variables need to persist and release unused references when possible.
javascript
Copy code
function createCounter() {
let count = 0;
return function() {
count++;
return count;
};
}
const counter = createCounter();
counter(); // 1
Break code into smaller functions: Modularizing your code into smaller, reusable functions helps manage scopes better. Each function should have a clear purpose, and this structure helps prevent variable clashes.
javascript
Copy code
function calculateArea(width, height) {
return width * height;
}
function calculateVolume(width, height, depth) {
return calculateArea(width, height) * depth;
}
Understand how hoisting works: Hoisting causes variable and function declarations to be moved to the top of their containing scope before the code executes. With var, this can lead to unexpected undefined values, while let and const declarations are not hoisted in the same way.
javascript
Copy code
console.log(a); // undefined, not an error
var a = 10;
console.log(b); // ReferenceError: Cannot access 'b' before initialization
let b = 10;
Activate strict mode: Enabling strict mode with "use strict"
; at the top of your JavaScript files ensures better scoping behavior. It disallows certain actions like using undeclared variables and makes your code more predictable.
javascript
Copy code
"use strict";
function example() {
undeclaredVar = 5; // ReferenceError: undeclaredVar is not defined
}
Remove unnecessary references: When working with closures or large data structures, clear out variables that are no longer needed to avoid memory leaks.
javascript
Copy code
function closureExample() {
let largeData = new Array(10000).fill('data');
return function() {
largeData = null; // Release memory
};
}
By following these practices, you can effectively manage lexical environments, reducing scoping issues and improving the maintainability of your JavaScript code.
Conclusion
Understanding lexical environments is crucial for writing clean, efficient JavaScript code. Lexical environments determine how variables are stored and accessed in different scopes, influencing how your code runs. By mastering this concept, developers can more easily debug issues like reference errors or unexpected variable access, particularly when dealing with nested functions and closures.
Being aware of how the scope chain works and how variables are resolved helps in structuring code more effectively. This ensures that variables are used appropriately, avoids common scoping pitfalls, and improves overall code readability and maintainability. Mastering lexical environments ultimately leads to fewer bugs, more predictable behavior, and optimized performance when working with JavaScript.
FAQs (from People Also Ask)
What is a lexical environment in simple terms?
How does lexical scoping differ from dynamic scoping in JavaScript?
What is the difference between a lexical environment and a closure?
In simple terms, a lexical environment is a container where JavaScript stores variables and functions during the execution of code. It keeps track of all the data (like variables and functions) defined in a specific scope, whether globally or within a function. Every time JavaScript runs a function or block, a new lexical environment is created to manage the variables in that scope(
DEV Community
)
Lexical scoping in JavaScript means that a variable's scope is determined by its location in the source code. JavaScript resolves variables by looking at the code structure, checking the current scope, then moving outward through parent scopes. In contrast, dynamic scoping (which JavaScript doesn't use) resolves variables based on the call stack or the sequence of function calls during runtime, not their position in the code.
MDN Web Docs
A lexical environment is the scope where variables are stored and accessed during execution. A closure, on the other hand, is a function that retains access to its outer lexical environment, even after the outer function has finished executing. Closures allow inner functions to "remember" variables from their parent environments, even when those environments are no longer active(
DEV Community
)(
DEV Community
).
Full Stack Development Courses in Different Cities
- Srinagar
- Bangalore
- Gujarat
- Haryana
- Punjab
- Delhi
- Chandigarh
- Maharashtra
- Tamil Nadu
- Telangana
- Ahmedabad
- Jaipur
- Indore
- Hyderabad
- Mumbai
- Agartala
- Agra
- Allahabad
- Amritsar
- Aurangabad
- Bhopal
- Bhubaneswar
- Chennai
- Coimbatore
- Dehradun
- Dhanbad
- Dharwad
- Faridabad
- Gandhinagar
- Ghaziabad
- Gurgaon
- Guwahati
- Gwalior
- Howrah
- Jabalpur
- Jammu
- Jodhpur
- Kanpur
- Kolkata
- Kota
- Lucknow
- Ludhiana
- Noida
- Patna
- Pondicherry
- Pune