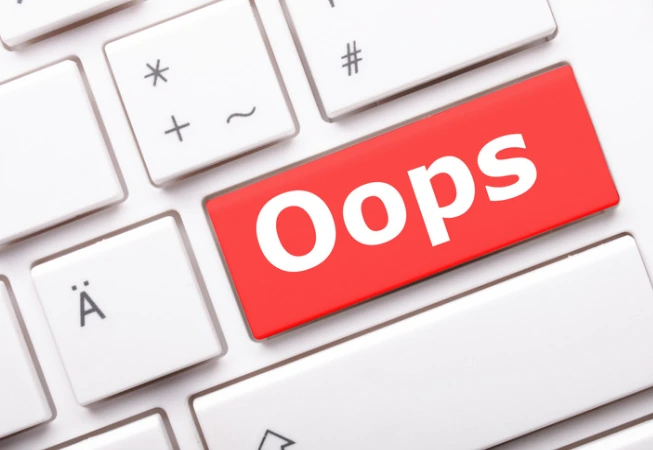
Brief Overview of Object-Oriented Programming (OOP)
Object-Oriented Programming, or OOP, is a programming method where data and functions are structured around objects. In OOP, each object represents a specific entity that holds both data and methods to operate on that data. This approach to programming makes it easier to model real-world situations in code as it allows you to create reusable objects that can interact in complex ways.
Importance of OOP in Modern Programming and Interviews
OOP is fundamental in software development and is often a key focus in interviews. Its principles are widely used because they improve the organization and maintainability of code. This makes OOP essential for large projects and collaborative work where consistent structure is crucial. Knowing OOP concepts is especially helpful for roles that involve languages like Java, Python, C++, and C#, which support OOP natively.
Key OOP Principles: Encapsulation, Abstraction, Inheritance, and Polymorphism
- Encapsulation: This concept involves bundling data and methods that operate on that data into a single unit or class. It helps keep the data safe from outside interference and misuse.
- Abstraction: Abstraction hides unnecessary details from users and exposes only what is relevant. By using abstraction, developers can focus on higher-level operations without getting bogged down by complex underlying details.
- Inheritance: Inheritance lets one class inherit properties and behaviors from another, allowing for code reuse and better organization. It simplifies how classes share attributes and methods.
- Polymorphism: Polymorphism allows objects to be treated as instances of their parent class. It lets the same method do different things based on the object calling it, enhancing flexibility in code.
Question Bank
What is Object-Oriented Programming (OOP)?
Why is OOP Important in Programming?
What Are the Main Principles of OOP?
- Encapsulation: Groups data and methods together to protect the data from direct access by other parts of the program.
- Abstraction: Exposes only relevant details to the user, hiding the complexity of the underlying code.
- Inheritance: Allows a class to inherit properties and methods from another class, promoting code reuse.
- Polymorphism: Lets objects of different classes be treated as objects of a common superclass, allowing a single method to be used in different ways.
Differences Between OOP and Procedural Programming?
- Structure: OOP organizes code around objects, while procedural programming uses functions and logic flows.
- Modularity: OOP is more modular, allowing code to be broken into self-contained objects. Procedural programming focuses on procedures that follow a sequence of operations.
- Data Handling: OOP binds data and methods together, whereas procedural programming often handles data and operations separately.
Definition and Differences Between a Class and an Object?
- A class is a template, while an object is a specific instance of that template.
- A class defines the properties and behaviors, whereas an object contains the actual values and can perform actions.
- Classes don't occupy memory until an object is created, but objects use memory for their specific data.
How Do You Create an Object from a Class?
What is the Role of a Constructor in a Class?
- In languages like Python, the constructor is defined as
__init__
. - In Java, it shares the same name as the class and does not have a return type.
What is the Purpose of a Destructor?
How Does Encapsulation Improve Code Security?
Method Overloading vs. Method Overriding: Differences?
- Method Overloading occurs when multiple methods in the same class have the same name but differ in the type or number of parameters. It allows methods to perform similar tasks with different types or numbers of inputs, which makes the code more versatile. Overloading is generally resolved at compile time.
- Method Overriding happens when a subclass provides a specific implementation for a method that is already defined in its superclass. The overriding method must have the same name, parameter list, and return type as the original. Overriding allows subclasses to define behaviors specific to their type, making them more flexible. This is typically resolved at runtime through dynamic binding.
How Does Abstraction Simplify Complex Systems?
What is a Virtual Function, and How is It Used?
Give definition and Usage of Abstract Classes
How Interfaces Differ from Abstract Classes?
- An interface can only contain method declarations (no implementation) and constants. It defines a contract that implementing classes must follow.
- An abstract class can have both fully implemented methods and abstract ones, offering more flexibility.
Exception Handling in OOP: Explanation of try, catch, and throw
- try: A block where code that might throw an exception is placed.
- catch: A block that handles the exception, specifying the type of error it can catch.
- throw: Used to signal an exception has occurred. When an exception is thrown, control is passed to the nearest catch block.
What is Operator Overloading, and Where is it Useful?
Explanation of Friend Functions in C++ and Their Purpose
Overview of Access Modifiers: Private, Protected, and Public
- Private: Members declared as private can only be accessed within the class itself. This is the default access level and is used to secure data, so it's not accessible from outside the class.
- Protected: Members declared as protected can be accessed within the class and by derived classes. Protected access is often used in inheritance to allow subclasses to access parent class data without exposing it to the rest of the program.
- Public: Members declared as public can be accessed from anywhere in the code where the object is visible. This modifier is typically used for methods or variables that need to be accessible to other parts of the program.
What are Manipulators in OOP?: Examples Like
endl and setw
What is a Constructor Overloading?
What is a Shallow Copy vs. Deep Copy?
What is a Singleton Class, and When Is It Used?
What is the Difference Between
this and super
Keywords?- The
this
keyword refers to the current object instance and is used within a class to access its own members, such as variables and methods. - The
super
keyword refers to the superclass (or parent class) and allows access to superclass members that the current class overrides. It's often used to call superclass constructors or methods from within a subclass.
Object-Oriented Programming (OOP) is a way of organizing code by structuring it around objects rather than just functions and logic. In OOP, objects are instances of classes, which act as templates defining the attributes and behaviors for a particular entity. OOP allows for modular design, meaning each object has its own data and methods, making it easier to develop, test, and maintain software.
OOP is important because it allows developers to create code that is organized, reusable, and adaptable. By using objects and classes, programmers can better model real-world situations in their code, making it more intuitive to understand and work with. OOP principles also promote collaboration as code can be more easily divided into independent parts. Additionally, concepts like encapsulation and inheritance improve security and enable efficient code reuse.
The four main principles of OOP are:
OOP and procedural programming take different approaches to organizing code:
A class is a blueprint or template that defines the properties (attributes) and behaviors (methods) for objects. In programming, it serves as the foundation for creating multiple instances of similar objects, each with its own set of values. Think of a class like a cookie cutter that shapes the cookies (objects), where each cookie can have different ingredients but follows the same overall shape.
An object is an instance of a class. When a class is defined, no memory is allocated until an object of that class is created. Each object has its own unique values for the attributes defined by the class, and it can perform the behaviors specified by the class's methods. For example, if "Car" is a class, then "myCar" could be an object of that class with specific attributes like color, model, and speed.
Key Differences:
To create an object, you need to declare it based on the class. The syntax varies by programming language but generally follows this format:
class Car:
def __init__(self, color, model):
self.color = color
self.model = model
# Creating an object
myCar = Car("Red", "Sedan")
In this example, myCar
is an object of the Car
class with the attributes color
set to "Red" and model
set to "Sedan".
A constructor is a special method that automatically initializes new objects of a class. When an object is created, the constructor is called to set up initial values for its attributes or to perform any setup actions necessary. Constructors ensure that each object starts in a consistent state.
For example:
public class Car {
private String color;
private String model;
// Constructor
public Car(String color, String model) {
this.color = color;
this.model = model;
}
}
A destructor is a method that cleans up resources when an object is no longer needed. In languages with automatic memory management (like Java or Python), explicit destructors aren't usually necessary, as the garbage collector handles it. However, in languages like C++, destructors are used to release resources like memory or open files to prevent memory leaks.
Encapsulation binds data and the methods that operate on that data into a single unit, often a class, and restricts access to some components. This improves security by preventing outside interference or unintended alterations to sensitive data. Only specific parts of the code can directly interact with the private data, while other parts must use public methods (getters and setters) provided by the class. This structure protects the integrity of the data and reduces the risk of accidental or malicious manipulation.
Abstraction hides the complex details of a system, exposing only the necessary parts to the user. By focusing on the essential aspects, abstraction allows programmers to work with high-level concepts without worrying about intricate details. For example, when using a car, a driver doesn't need to know how the engine works—just how to start, stop, and steer the car. In coding, abstraction simplifies development, making it easier to build and maintain complex systems by breaking them into smaller, more manageable parts.
A virtual function is a member function in a base class that can be overridden in a derived class. Virtual functions allow polymorphism, meaning a pointer to the base class can invoke methods of derived classes, depending on the object's actual type at runtime. This flexibility is particularly useful in scenarios where different classes share common behavior but may have unique implementations. Virtual functions are declared using the virtual
keyword in C++
and are commonly used for dynamic binding.
An abstract class is a class that cannot be instantiated on its own and often contains one or more abstract methods—methods declared without a concrete implementation. Abstract classes serve as base classes that other classes inherit, providing a common structure while requiring derived classes to implement specific behaviors. For instance, an abstract class Shape might include an abstract method calculateArea()
, which must be defined by subclasses like Circle and Rectangle
.
Both interfaces and abstract classes define methods for subclasses to implement, but they have some key differences:
Interfaces support multiple inheritance, meaning a class can implement multiple interfaces. Abstract classes, however, allow a single inheritance chain.
Exception handling manages runtime errors in a program to prevent crashes. It uses the following constructs:
Operator overloading allows you to redefine or “overload” operators (like +, -, *, etc.) so they can work with user-defined data types (such as classes). This feature enables operators to perform operations on objects in the same way they would on primitive data types.
For example, if you have a Complex class for complex numbers, you can overload the + operator to add two complex numbers:
class Complex {
public:
int real, imag;
Complex operator + (const Complex& obj) {
Complex temp;
temp.real = real + obj.real;
temp.imag = imag + obj.imag;
return temp;
}
};
Operator overloading is useful because it makes code more intuitive and readable, allowing for operations that feel natural when dealing with objects. This feature is common in C++ and makes custom data types more flexible and expressive.
A friend function in C++ is a function that has special access to the private and protected members of a class, even though it is not a member of the class itself. Friend functions are declared by adding the friend keyword in front of the function declaration inside the class.
For example:
class Box {
private:
int width;
public:
friend void setWidth(Box &b, int w);
};
void setWidth(Box &b, int w) {
b.width = w; // Accessing private member width directly
}
Friend functions are useful when two or more classes need to work closely together. They allow functions to access otherwise restricted data without making all data public. This keeps class internals private while still permitting controlled access.
Access modifiers in C++ and other OOP languages control the visibility and accessibility of class members:
These modifiers help maintain data integrity by restricting access based on the needs of the program and the design of the classes.
Manipulators in C++ are special functions that change the format of output. They are used with insertion (<<)
or extraction (>>)
operators to control the display of data.
endl:
This manipulator is used to insert a newline character and flush the output buffer. It's commonly used to end a line of output.
setw:
Part of the iomanip
library, setw
sets the width for the next item output. It is often used for formatting tables or aligning output text.
Manipulators improve readability and structure in output, making data presentation more organized and easier to understand.
Constructor overloading is when a class has multiple constructors with different parameters. Each constructor can initialize objects differently, depending on the parameters passed. This is useful for creating objects in various ways while still using the same class.
What is Multiple Inheritance, and Does Java Support It? Multiple inheritance allows a class to inherit from more than one base class. While C++ supports multiple inheritance directly, Java does not, as it could lead to complexity and ambiguity (often called the "diamond problem"). Instead, Java allows classes to implement multiple interfaces to achieve similar behavior.
A shallow copy duplicates only the top-level objects, whereas a deep copy duplicates objects at all levels. In a shallow copy, if the original object contains references to other objects, both copies will refer to the same object instances. In contrast, a deep copy will create new instances of each object referred to by the original.
A singleton class is a design pattern that restricts a class to only one instance and provides a global access point to that instance. Singleton classes are useful when only one instance is needed to manage system-wide resources, such as a configuration manager or logging utility.
Conclusion
Recap of OOP Importance in Programming Interviews
Object-Oriented Programming (OOP) is a cornerstone in software development, making it essential for candidates to understand when applying for programming positions. Many programming languages use OOP principles like encapsulation, inheritance, and polymorphism to organize code efficiently. This structure is critical in interviews as it demonstrates a candidate's ability to design modular, reusable, and maintainable software. Mastery of OOP shows a thorough understanding of how to build systems that reflect real-world entities and their interactions.
Tips for Answering OOP Questions Effectively
- Understand the Basics: Be clear on foundational concepts such as classes, objects, encapsulation, and polymorphism. Interviewers often focus on these principles to gauge a candidate's grasp of OOP fundamentals.
- Use Real-World Analogies: Relating complex OOP concepts to everyday objects can make your answers more relatable and understandable. For example, you might describe a class as a blueprint for creating objects, much like a cookie cutter creates cookies.
- Explain Without Code: While knowing how to implement concepts in code is essential, it's equally important to explain the logic behind the concepts. This shows you understand why and when to use OOP structures.
- Ask Clarifying Questions: If an OOP question isn't entirely clear, ask for clarification. It demonstrates your ability to approach problems methodically and ensures you're providing the most relevant answer.
- Practice Common Patterns: Familiarize yourself with OOP design patterns like Singleton or Factory as they often come up in interviews. Understanding these patterns will help you discuss their use cases confidently.
Additional Resources for Learning OOP Concepts
- Books: Head First Object-Oriented Analysis and Design by Brett McLaughlin and Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma are excellent for building a solid foundation in OOP and understanding design patterns.
- Online Courses: Websites like Coursera and edX offer courses on OOP in multiple programming languages, providing both beginner and advanced options.
Full Stack Development Courses in Different Cities
- Srinagar
- Bangalore
- Gujarat
- Haryana
- Punjab
- Delhi
- Chandigarh
- Maharashtra
- Tamil Nadu
- Telangana
- Ahmedabad
- Jaipur
- Indore
- Hyderabad
- Mumbai
- Agartala
- Agra
- Allahabad
- Amritsar
- Aurangabad
- Bhopal
- Bhubaneswar
- Chennai
- Coimbatore
- Dehradun
- Dhanbad
- Dharwad
- Faridabad
- Gandhinagar
- Ghaziabad
- Gurgaon
- Guwahati
- Gwalior
- Howrah
- Jabalpur
- Jammu
- Jodhpur
- Kanpur
- Kolkata
- Kota
- Lucknow
- Ludhiana
- Noida
- Patna
- Pondicherry
- Pune